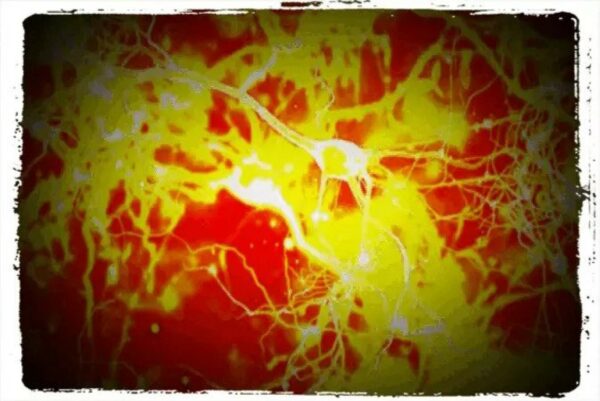
TensorFlow is an open-source machine learning framework developed by Google Brain Team, which allows developers to build, train and deploy machine learning models at scale. TensorFlow was first introduced in November 2015, and since then, it has become one of the most widely used machine learning libraries in the industry.
In this article, we will cover the basics of TensorFlow and demonstrate how to use it to build a simple machine learning model. We will provide code snippets throughout the article, and at the end, we will include a step-by-step tutorial on how to build a basic model with TensorFlow.
What is TensorFlow?
TensorFlow is a library for numerical computation using data flow graphs. It was designed to be a flexible and scalable platform for building and training machine learning models. In TensorFlow, computations are expressed as graphs, where nodes represent mathematical operations, and edges represent the data flowing between them.
TensorFlow provides a high-level API for building neural networks, called Keras, which makes it easy to construct and train complex models. TensorFlow also provides low-level APIs for building custom models and implementing new algorithms.
TensorFlow is written in C++ and provides APIs for Python, Java, C++, and other languages. The Python API is the most widely used and provides an easy-to-use interface for building machine learning models.
Getting Started with TensorFlow
To get started with TensorFlow, you first need to install it. The easiest way to install TensorFlow is through pip, which is a package manager for Python. To install TensorFlow, run the following command:
pip install tensorflow
Once you have installed TensorFlow, you can import it into your Python code using the following command:
import tensorflow as tf
TensorFlow Variables and Operations
In TensorFlow, variables are used to store the state of the model during training. Variables are defined using the tf.Variable() function and can be initialized to a specific value or randomly initialized. Operations are used to perform mathematical computations on variables and other data. In TensorFlow, operations are defined using the tf.Operation() function.
Here is an example of how to create a variable and perform an operation on it:
import tensorflow as tf
# create a variable with an initial value of 2
x = tf.Variable(2.0, dtype=tf.float32)
# create an operation that multiplies x by 3
y = tf.multiply(x, 3)
# create a session to run the operation
with tf.Session() as sess:
# initialize the variable
sess.run(tf.global_variables_initializer())
# evaluate the operation and print the result
result = sess.run(y)
print(result)
In this example, we create a variable x with an initial value of 2 and an operation y that multiplies x by 3. We then create a session to run the operation and evaluate the result.
Building a Simple Machine Learning Model with TensorFlow
Now that we have covered the basics of TensorFlow, let’s build a simple machine learning model. In this example, we will use the Boston Housing dataset, which contains information about housing prices in Boston. Our goal is to build a model that can predict the median value of owner-occupied homes in Boston.
Here is the code to load the Boston Housing dataset and preprocess it:
import tensorflow as tf
from sklearn.datasets import load_boston
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# load the Boston Housing dataset
boston = load_boston()
# split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(
boston.data, boston.target, test_size=0.2, random_state=0)
# normalize the features using StandardScaler
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
We first import TensorFlow, as well as the Boston Housing dataset from scikit-learn. We then split the dataset into training and testing sets using train_test_split()
and normalize the features using StandardScaler()
.
Next, we will build our machine learning model using TensorFlow. Our model will have one hidden layer with 10 neurons and a ReLU activation function. We will use mean squared error as our loss function and the Adam optimizer to minimize the loss.
# define the hyperparameters
learning_rate = 0.01
num_epochs = 100
batch_size = 32
# define the number of features and the number of output classes
num_features = X_train.shape[1]
num_classes = 1
# define the placeholders for the input and output data
X = tf.placeholder(tf.float32, [None, num_features])
y = tf.placeholder(tf.float32, [None, num_classes])
# define the weights and biases for the hidden layer
W1 = tf.Variable(tf.random_normal([num_features, 10]))
b1 = tf.Variable(tf.random_normal([10]))
# define the output of the hidden layer
hidden_out = tf.nn.relu(tf.add(tf.matmul(X, W1), b1))
# define the weights and biases for the output layer
W2 = tf.Variable(tf.random_normal([10, num_classes]))
b2 = tf.Variable(tf.random_normal([num_classes]))
# define the output of the output layer
y_pred = tf.add(tf.matmul(hidden_out, W2), b2)
# define the loss function
loss = tf.reduce_mean(tf.square(y_pred - y))
# define the optimizer
optimizer = tf.train.AdamOptimizer(learning_rate).minimize(loss)
In this code, we define the hyperparameters for our model, such as the learning rate, number of epochs, and batch size. We also define the number of features and output classes.
We then define the placeholders for the input and output data using tf.placeholder(). We define the weights and biases for the hidden and output layers using tf.Variable(), and we use the ReLU activation function for the hidden layer. We also define the loss function and the optimizer using tf.reduce_mean() and tf.train.AdamOptimizer().
Finally, we will train our model using the training data and evaluate it using the testing data. Here is the code to do this:
# create a session to run the model
with tf.Session() as sess:
# initialize the variables
sess.run(tf.global_variables_initializer())
# train the model for the specified number of epochs
for epoch in range(num_epochs):
epoch_loss = 0
# loop over the training data in batches
for i in range(0, len(X_train), batch_size):
# get a batch of training data and labels
batch_x = X_train[i:i+batch_size]
batch_y = y_train[i:i+batch_size]
# run the optimizer and calculate the loss
_, batch_loss = sess.run([optimizer, loss], feed_dict={X: batch_x, y: batch_y})
# accumulate the loss for the epoch
epoch_loss += batch_loss
# print the average loss for the epoch
print('Epoch', epoch, 'loss:', epoch_loss/len(X_train))
# evaluate the model on the testing data
y_pred_test = sess.run(y_pred, feed_dict={X: X_test})
We first create a TensorFlow session using with tf.Session() as sess:. We then initialize the variables using sess.run(tf.global_variables_initializer()).
We train the model for the specified number of epochs, and for each epoch we loop over the training data in batches. We use sess.run() to run the optimizer and calculate the loss for each batch.
After each epoch, we print the average loss. Finally, we evaluate the model on the testing data using sess.run() and store the predicted output in y_pred_test.
We can calculate the mean squared error of the predictions using scikit-learn:
# calculate the mean squared error of the predictions
mse = mean_squared_error(y_test, y_pred_test)
print('Mean squared error:', mse)
Now that we have seen how to build and train a simple machine learning model in TensorFlow, we can explore more advanced techniques and architectures.
Here are some additional topics that you can explore:
Convolutional neural networks (CNNs) for image classification
Recurrent neural networks (RNNs) for natural language processing (NLP)
Transfer learning using pre-trained models
Hyperparameter tuning using grid search or random search
Overall, TensorFlow is a powerful and flexible machine learning framework that is widely used in industry and academia. With its high-level APIs and extensive documentation, it is easy to get started with TensorFlow and to build and train your own machine learning models.
Youtube / Video Tutorials
Here are some recommended YouTube lectures on TensorFlow:
- TensorFlow YouTube Channel: The official TensorFlow YouTube channel provides a variety of videos on TensorFlow, including tutorials, live streams, and talks from TensorFlow events. The channel covers a wide range of topics, from beginner-level introductions to advanced techniques.
- TensorFlow 2.0 Beginner Tutorials by Sentdex: This video series by Sentdex provides a series of beginner-level tutorials on TensorFlow 2.0. The series covers topics such as building and training neural networks, using TensorFlow with Keras, working with image and text data, and using TensorFlow for transfer learning.
- TensorFlow 2.0 Complete Course – Python Neural Networks for Beginners Tutorial: This video provides a complete course on TensorFlow 2.0 for beginners. The video covers topics such as installing TensorFlow, building and training models, and evaluating performance. The course is taught by freeCodeCamp.org and provides hands-on coding exercises to help learners build their own models.
- TensorFlow 2.0 Tutorial for Deep Learning: This video by Krish Naik provides a tutorial on TensorFlow 2.0 for deep learning. The video covers topics such as building a neural network, using convolutional layers for image recognition, and using recurrent layers for natural language processing. The video also provides coding examples for each topic.
Reference Documentation and Forums
Here are some technical references for TensorFlow:
- TensorFlow Official Documentation – The official documentation provides comprehensive guides, API references, tutorials, and examples for TensorFlow.
- TensorFlow GitHub Repository – The TensorFlow GitHub repository provides the source code for TensorFlow, including examples and tutorials.
- TensorFlow White Paper – The TensorFlow white paper provides a technical overview of TensorFlow, including its design, architecture, and features.
- “TensorFlow: A System for Large-Scale Machine Learning” – This paper by Google researchers provides a detailed technical description of TensorFlow’s design and implementation.
- “Deep Learning with TensorFlow 2 and Keras” – This book by Antonio Gulli and Amita Kapoor provides a comprehensive guide to building and training deep learning models using TensorFlow 2 and Keras.
- “Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow” – This book by Aurélien Géron provides a hands-on guide to building and training machine learning models using TensorFlow and Keras.
- “TensorFlow for Deep Learning” – This book by Bharath Ramsundar and Reza Bosagh Zadeh provides a technical guide to building and training deep learning models using TensorFlow.
These technical references provide a deeper understanding of TensorFlow’s design, implementation, and features, and they are useful for developers and researchers who want to build and optimize their own machine learning models using TensorFlow.
Reference Technical Books
Here are some popular technical books on TensorFlow:
- Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow, 2nd Edition by Aurélien Géron: This book provides a practical guide to machine learning with TensorFlow and Keras, covering topics such as building and training neural networks, using convolutional and recurrent networks for image and language processing, and deploying models to production. The book also covers best practices for hyperparameter tuning, model evaluation, and data preprocessing.
- TensorFlow 2.0 Cookbook by Antonio Gulli, Amita Kapoor, and Sujit Pal: This book provides a collection of recipes for building and training machine learning models with TensorFlow 2.0, covering topics such as data preprocessing, image and text processing, natural language processing, and reinforcement learning. The book also covers best practices for debugging and optimizing models, and deploying models to production.
- TensorFlow for Deep Learning: From Linear Regression to Reinforcement Learning by Bharath Ramsundar and Reza Bosagh Zadeh: This book provides a comprehensive introduction to deep learning with TensorFlow, covering topics such as building and training neural networks, using convolutional and recurrent networks for image and language processing, and applying deep learning to real-world problems such as recommendation systems and fraud detection. The book also covers best practices for model evaluation, debugging, and optimization.
- Hands-On Deep Learning Algorithms with TensorFlow by Santanu Pattanayak and Md. Rezaul Karim: This book provides a hands-on guide to deep learning with TensorFlow, covering topics such as building and training neural networks, using convolutional and recurrent networks for image and language processing, and applying deep learning to real-world problems such as self-driving cars and speech recognition. The book also covers best practices for model evaluation, debugging, and optimization.
These books are useful for developers and researchers who want to learn how to build and train their own machine learning models using TensorFlow. They provide practical guidance and best practices for working with TensorFlow, from beginner-level introductions to advanced techniques.
Cheat Sheet
TensorFlow Cheat Sheet by GitHub: This cheat sheet provides a quick reference guide to TensorFlow, covering topics such as building and training neural networks, using convolutional and recurrent networks for image and language processing, and applying deep learning to real-world problems. The cheat sheet also includes sample code snippets and best practices for model evaluation, debugging, and optimization.
Conclusion
In conclusion, TensorFlow is a powerful open-source machine learning library that enables developers and researchers to build and train their own machine learning models. It provides a flexible and scalable platform for working with deep learning models, and has a wide range of applications in areas such as image and language processing, natural language processing, and reinforcement learning. With the resources available such as technical books, YouTube videos, and cheat sheets, developers and researchers can learn how to use TensorFlow effectively and efficiently. As the field of machine learning continues to grow and evolve, TensorFlow will remain a key tool for developing and deploying deep learning models.