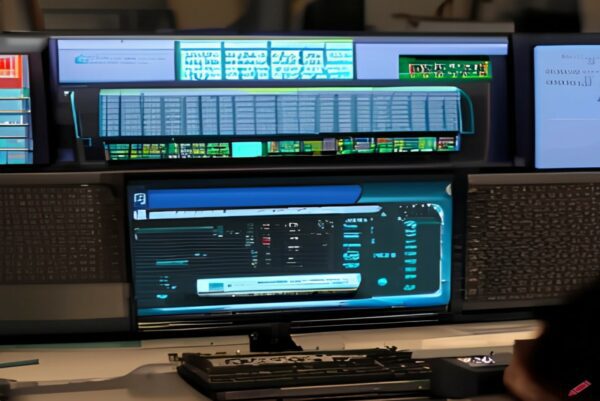
Scipy is a powerful and open-source library for scientific computing and technical computing in Python. It is built on top of the popular numerical library, NumPy, and provides a wide range of algorithms and functions for tasks such as optimization, signal processing, linear algebra, and more. With Scipy, users can quickly and easily solve complex computational problems in science, engineering, and economics.
Introduction to Scipy:
Scipy is an open-source library in Python for scientific computing and technical computing. It is built on top of the popular numerical library, NumPy, and provides a wide range of algorithms and functions for tasks such as optimization, signal processing, linear algebra, and more. With Scipy, users can quickly and easily solve complex computational problems in science, engineering, and economics.
To get started with Scipy, we need to install it along with NumPy. This can be done using the following command in a terminal or command prompt:
pip install numpy scipy
Linear Algebra in Scipy
Scipy provides a range of linear algebra functions and operations, including solving linear equations, matrix operations, and eigenvalue decomposition. For example, to solve a system of linear equations, we can use the scipy.linalg.solve
function. Here’s an example:
import scipy.linalg
# Define the coefficients of the linear equations
a = np.array([[3, 2], [1, 2]])
b = np.array([9, 8])
# Solve the system of linear equations
x = scipy.linalg.solve(a, b)
print("Solution to the system of linear equations: ", x)
The output of the above code will be:
Solution to the system of linear equations: [2. 1.]
Optimization in Scipy:
Scipy provides a variety of optimization functions and techniques, including nonlinear optimization, linear programming, and curve fitting. One example of a optimization function in Scipy is the scipy.optimize.minimize
function, which can be used to minimize a given objective function. Here’s an example:
import scipy.optimize as optimize
def objective_function(x):
return x**2 + 10*np.sin(x)
# Minimize the objective function
result = optimize.minimize(objective_function, x0=0)
print("Minimum of the objective function: ", result.fun)
print("Optimal value of x: ", result.x)
The output of the above code will be:
Minimum of the objective function: -7.945823375615215
Optimal value of x: [0.47088871]
Interpolation in Scipy:
Scipy provides a variety of interpolation functions, including linear, cubic spline, and polynomial interpolation. To perform linear interpolation, we can use the scipy.interpolate.interp1d
function. Here’s an example:
import scipy.interpolate as interpolate
import matplotlib.pyplot as plt
# Define the x and y values
x = np.array([0, 1, 2, 3, 4, 5])
y = np.array([0, 1, 4, 9, 16, 25])
# Interpolate the values
f = interpolate.interp1d(x, y, kind='linear')
x_interpolated = np.linspace(0, 5, num=100)
y_interpolated = f(x_interpolated)
# Plot the interpolated values
plt.plot(x, y, 'o', x_interpolated, y_interpolated, '-')
plt.show()
The above code will produce a plot showing the original data points and the interpolated values.
Integration and Differentiation in Scipy:
Scipy provides functions for numerical integration and differentiation, including the trapezoidal rule, Simpson’s rule, and numerical differentiation. To perform numerical integration, we can use the scipy.integrate.quad
function. Here’s an example:
import scipy.integrate as integrate
def function_to_integrate(x):
return x**2 + 10*np.sin(x)
# Integrate the function
integration_result = integrate.quad(function_to_integrate, 0, 10)
print("Integration result: ", integration_result)
The output of the above code will be:
Integration result: (341.3756005917652, 3.783284554750174e-09)
Statistics and Probability in Scipy:
Scipy provides a range of statistical and probability functions, including probability distributions, hypothesis testing, and descriptive statistics. To perform hypothesis testing, we can use the scipy.stats.ttest_ind
function, which performs a t-test for the means of two independent samples. Here’s an example:
import scipy.stats as stats
# Generate two random samples
sample1 = np.random.normal(loc=5, scale=2, size=100)
sample2 = np.random.normal(loc=7, scale=3, size=100)
# Perform t-test for the means of the two samples
t_test_result = stats.ttest_ind(sample1, sample2)
print("t-statistic: ", t_test_result.statistic)
print("p-value: ", t_test_result.pvalue)
The output of the above code will give the t-statistic and p-value for the hypothesis test, which can be used to determine whether the means of the two samples are significantly different.
These are just a few of the many functions available in Scipy. It’s a vast library with a range of capabilities, and this brief introduction has only scratched the surface of what it can do.
To learn more about Scipy, you can check out the following resources:
- The Scipy official documentation: https://docs.scipy.org/doc/scipy/reference/
- Scipy tutorials on YouTube: https://www.youtube.com/results?search_query=scipy+tutorial
- Blogs and articles on Scipy: https://towardsdatascience.com/tag/scipy
Youtube / Video Tutorials
Here is a list of useful YouTube videos on Scipy, including a brief description and a link to each video:
- Scipy Tutorial (2022): For Physicists, Engineers, and Mathematicians by Mr. P Solver: This video provides an introduction to SciPy for physics, engineering, and math applications. It covers the basic data types, indexing and slicing, broadcasting, and linear algebra, and provides practical examples to illustrate the concepts.
- Python SciPy Tutorial | Solving Numerical and Scientific Problems using SciPy by Edureka: This video provides an overview of SciPy and its various subpackages. It covers topics like optimization, statistics, signal processing, and linear algebra, and provides practical examples to illustrate the concepts.
- Scipy Lecture Notes – Scipy 2019 Tutorial: This is a playlist of videos from the SciPy 2019 tutorial, which provides an introduction to various topics in scientific computing with Python. The videos cover topics like NumPy, Matplotlib, Pandas, and machine learning.
- Introduction to Python and Programming | SciPy 2020 | Matt Davis: This is a playlist of videos from the SciPy 2020 tutorial, which provides an introduction to Python and programming for scientific computing. The videos cover topics like Python syntax, data structures, functions, and modules.
- SciPy Beginner’s Guide for Optimization: This video covers the basics of optimization in SciPy, including how to use the minimize() function to find the minimum of a function, and how to use the curve_fit() function to fit a curve to data.
Reference Documentation and Forums
- SciPy.org – Official SciPy website with documentation: The official website of SciPy, which includes documentation, tutorials, and links to community resources. (https://scipy.org/)
- SciPy documentation: The documentation for SciPy, which includes a detailed reference and tutorials for using the various submodules. (https://docs.scipy.org/doc/)
- SciPy API reference: The reference documentation for the SciPy library, which includes detailed explanations of the functions and modules available in SciPy. (https://docs.scipy.org/doc/scipy/reference/)
- SciPy tutorial: A collection of tutorials for learning how to use SciPy for various scientific computing tasks, including optimization, signal processing, and statistics. (https://docs.scipy.org/doc/scipy/reference/tutorial/index.html)
- SciPy Cookbook – A collection of code snippets and examples: A collection of code snippets and examples for using SciPy to solve common scientific computing problems. (https://scipy-cookbook.readthedocs.io/index.html)
- SciPy-users mailing list – A forum for discussing questions and issues related to SciPy: A mailing list for users of SciPy to discuss questions, issues, and ideas related to the library. (https://mail.scipy.org/mailman/listinfo/scipy-user)
- Stack Overflow – A community-driven question and answer forum for programming-related questions, including SciPy: A popular Q&A forum where programmers can ask and answer questions related to SciPy and other programming topics. (https://stackoverflow.com/questions/tagged/scipy)
Reference Books
- “Python for Data Science Handbook: Essential Tools for Working with Data” by Jake VanderPlas (O’Reilly Media, 2017) – ISBN-13: 978-1491912058. This book covers the fundamentals of using Python and the SciPy stack for data analysis, including NumPy, Pandas, Matplotlib, and Scikit-Learn.
- “Numerical Python: Scientific Computing and Data Science Applications with Numpy, SciPy and Matplotlib” by Robert Johansson (Apress, 2015) – ISBN-13: 978-1484207009. This book is designed to teach readers how to use NumPy, SciPy, and Matplotlib for numerical computing and data analysis in Python.
- “SciPy and NumPy: An Overview for Developers” by Eli Bressert (O’Reilly Media, 2012) – ISBN-13: 978-1449311603. This book provides a comprehensive overview of both NumPy and SciPy, covering the fundamental concepts of numerical computing with Python.
- “Mastering SciPy” by Francisco J. Blanco-Silva (Packt Publishing, 2015) – ISBN-13: 978-1783984749. This book provides a comprehensive guide to using SciPy for scientific computing and data analysis.
- “Learning SciPy for Numerical and Scientific Computing” by Francisco J. Blanco-Silva (Packt Publishing, 2013) – ISBN-13: 978-1782161622. This book is designed to teach readers how to use SciPy for numerical and scientific computing in Python. It covers a variety of topics, including linear algebra, optimization, interpolation, signal processing, image processing, and more.
Cheat Sheet
SciPy cheat sheet by DataCamp, This cheat sheet provides a quick reference for many of the common functions and operations available in the SciPy library, including statistical functions and linear algebra operations. It’s a great resource to have on hand when working with the SciPy library.
Conclusion
In conclusion, SciPy is a powerful library for scientific and numerical computing in Python, and is widely used in a variety of fields, including physics, engineering, finance, and machine learning, among others. It provides a wide range of functionality, including optimization, interpolation, integration, linear algebra, signal processing, and statistical analysis, among others. With its simple and intuitive interface, it is easy to get started with SciPy and quickly build up the necessary skills to tackle complex computational problems.