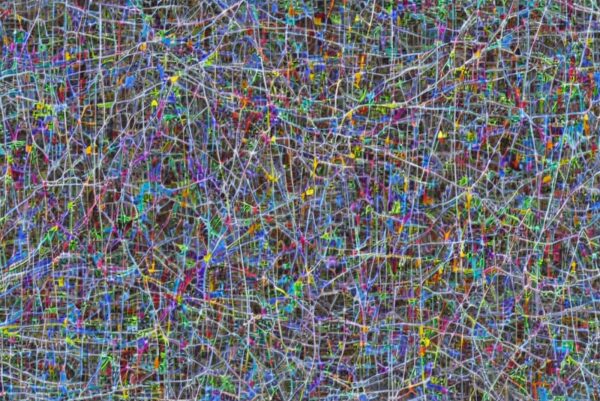
Introduction
PyTorch is an open-source machine learning library that is widely used in deep learning research and production. It was developed by Facebook’s AI Research lab and released in 2016. PyTorch is known for its ease of use, flexibility, and dynamic computational graph, which make it a popular choice among researchers and practitioners.
One of the main features of PyTorch is its dynamic computational graph, which allows for efficient and flexible computation of gradients. Unlike some other deep learning libraries, PyTorch uses a dynamic computational graph, which means that the graph is constructed on the fly during computation, rather than being predefined. This makes it easy to modify the network architecture on the fly, which is useful for researchers who want to experiment with different network structures.
PyTorch also provides a variety of tools for building deep learning models, including automatic differentiation, an extensive library of pre-built modules, and a suite of tools for building custom modules. PyTorch supports a range of popular neural network architectures, including convolutional neural networks (CNNs), recurrent neural networks (RNNs), and transformers. In addition, PyTorch has a large and active community of users, which means that there are many resources available for learning and using the library.
Overall, PyTorch is a powerful and flexible deep learning library that is widely used in research and production. Its dynamic computational graph, ease of use, and flexible API make it a popular choice for researchers and practitioners who want to build and experiment with deep learning models.
Installing PyTorch
To get started with PyTorch, you need to install it. You can install PyTorch by running the following command:
pip install torch torchvision
This will install PyTorch and torchvision, which is a PyTorch package that provides access to popular datasets, such as MNIST and CIFAR-10.
PyTorch Basics
To create a tensor in PyTorch, you can use the torch.tensor() function. Here’s an example:
import torch
# Create a tensor
x = torch.tensor([1, 2, 3])
# Print the tensor
print(x)
Output:
tensor([1, 2, 3])
Building a Neural Network in PyTorch
To build a neural network in PyTorch, you need to define a class that inherits from the torch.nn.Module class. Here’s an example:
import torch.nn as nn
class MyNet(nn.Module):
def __init__(self):
super(MyNet, self).__init__()
self.fc1 = nn.Linear(784, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = x.view(-1, 784)
x = nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
In this example, we define a neural network with two fully connected layers. The first layer takes an input of size 784 (the size of a flattened MNIST image) and outputs a tensor of size 128. The second layer takes an input of size 128 and outputs a tensor of size 10 (the number of classes in MNIST).
Training a Neural Network in PyTorch
To train a neural network in PyTorch, you need to define a loss function and an optimizer. Here’s an example:
import torch.optim as optim
net = MyNet()
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(net.parameters(), lr=0.01, momentum=0.9)
# Train the network
for epoch in range(10):
running_loss = 0.0
for i, data in enumerate(trainloader, 0):
inputs, labels = data
optimizer.zero_grad()
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print('[%d] loss: %.3f' % (epoch + 1, running_loss / len(trainloader)))
Youtube / Video Tutorials
Here are some recommended YouTube lectures on PyTorch:
- “Learn PyTorch for Deep Learning in a Day. Literally.” by Daniel Bourke is a great resource for beginners who want to learn PyTorch. In this video, Bourke provides a concise and accessible introduction to PyTorch, covering key concepts such as tensors, autograd, and neural networks.
- “Deep Learning with PyTorch – Full Course” by Patrick Loeber is a comprehensive course that covers the fundamentals of deep learning and how to implement them using PyTorch. This course is designed for beginners who have little or no experience with PyTorch or deep learning.
- “PyTorch Beginner Series” is a collection of tutorials created by the PyTorch team that are specifically designed for beginners who want to learn PyTorch. The series covers a wide range of topics, from the basics of PyTorch to more advanced concepts such as deep learning and computer vision.
- “PyTorch for Deep Learning & Machine Learning – Full Course” by freecodecamp is a comprehensive course that covers the basics of PyTorch and how to use it for deep learning and machine learning. The course is designed for beginners who have little or no experience with PyTorch or deep learning.
Reference Documentation and Forums
Here are some technical references on PyTorch:
- PyTorch official documentation: PyTorch’s official documentation is a comprehensive resource that provides information on installation, usage, and development with PyTorch. It includes tutorials, API reference, and examples of different applications.
- PyTorch tutorials: The PyTorch website also provides a series of tutorials that cover a range of topics from the basics of tensors to advanced topics such as Generative Adversarial Networks (GANs) and Natural Language Processing (NLP).
- “Deep Learning with PyTorch” book by Eli Stevens, Luca Antiga, and Thomas Viehmann: This book is a comprehensive guide to PyTorch that covers the fundamentals of deep learning, PyTorch, and practical applications of deep learning.
- “Programming PyTorch for Deep Learning” book by Ian Pointer: This book is a practical guide to building deep learning models using PyTorch. It covers topics such as data preparation, training models, and deploying models to production.
- “PyTorch Recipes: A Problem-Solution Approach” book by Pradeepta Mishra and others: This book provides a collection of recipes that solve common problems faced by deep learning practitioners using PyTorch. It covers topics such as data processing, model selection, and model optimization.
- “PyTorch Lightning” documentation: PyTorch Lightning is a lightweight PyTorch wrapper that provides a simplified interface for building deep learning models. The PyTorch Lightning documentation provides information on installation, usage, and development with PyTorch Lightning.
These are just a few examples of the many technical references available on PyTorch. PyTorch has a large and active community, which means there are many resources available to help you learn and build your deep learning models.
Reference Technical Books
- “Deep Learning with PyTorch” by Eli Stevens, Luca Antiga, and Thomas Viehmann, published by Manning Publications. This book is an excellent introduction to deep learning and PyTorch, covering the basics of PyTorch and building and training deep neural networks. The book is suitable for beginners and intermediate learners and includes code examples and practical exercises. ISBN: 9781617295263.
- “PyTorch Recipes: A Problem-Solution Approach” by Pradeepta Mishra and Sean Robertson, published by Apress. This book provides practical solutions to common problems faced by PyTorch users. The book covers topics such as data preprocessing, building neural networks, and deploying models to production. The book is suitable for intermediate and advanced users and includes code examples and practical exercises. ISBN: 9781484251423.
- “Programming PyTorch for Deep Learning: Creating and Deploying Deep Learning Applications” by Ian Pointer, published by O’Reilly Media. This book covers the fundamentals of deep learning and PyTorch, as well as how to create and deploy deep learning applications. The book is suitable for beginners and intermediate learners and includes code examples and practical exercises. ISBN: 9781492045359.
- “PyTorch: Advanced Tutorials” by PyTorch Contributors, published by PyTorch. This book is a collection of advanced tutorials on PyTorch, covering topics such as natural language processing, computer vision, and generative adversarial networks (GANs). The book is suitable for advanced users and includes code examples and practical exercises. ISBN: N/A.
- “Data Science Projects with PyTorch” by Manpreet Singh Ghotra and Shubham Gupta, published by Packt Publishing. This book covers how to apply PyTorch to data science projects, including data preprocessing, feature engineering, and building predictive models. The book is suitable for intermediate and advanced learners and includes code examples and practical exercises. ISBN: 9781801078247.
Cheat Sheet
The PyTorch Cheat Sheet is an excellent resource for anyone who wants to learn PyTorch or needs a quick reference guide to PyTorch commands and functions. It is available for free on the PyTorch website
Conclusion
In conclusion, PyTorch is a powerful deep learning framework that has gained popularity due to its ease of use, flexibility, and community support. It provides an intuitive and flexible interface for building and training deep neural networks and supports a wide range of applications, including computer vision, natural language processing, and reinforcement learning.