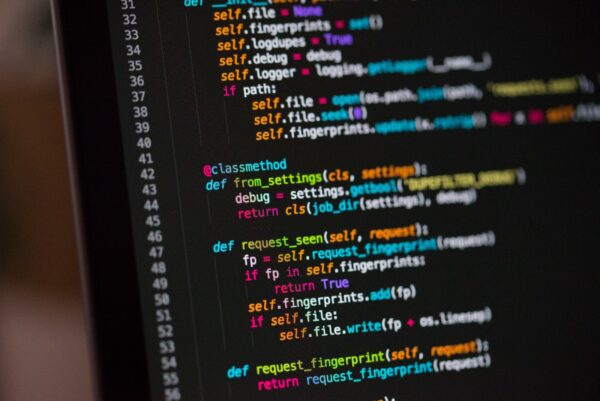
Python is a widely used high-level programming language that is popular for web development, machine learning, and data analysis. In this article, we’ll go over the basics of Python and provide resources to help you get started.
Introduction to Python
Python is known for its simple, readable syntax and its wide range of applications. It uses whitespace to delimit code blocks, making it easier to read than other programming languages that use braces or other characters. Please refer to Installation and Introductory Guide to Anaconda: A Python Distribution to setup environment.
Here’s a simple example of a Python program that outputs “Hello, World!” to the console:
print("Hello, World!")
Python has several built-in data types, including numbers (integers, floating-point numbers), strings, lists, dictionaries, and others. For example:
# Numbers
x = 42
y = 7.0
# Strings
name = "Alice"
greeting = "Hello, " + name + "!"
# Lists
fruits = ["apple", "banana", "cherry"]
# Dictionaries
person = {
"name": "Bob",
"age": 30,
"hobbies": ["reading", "traveling"]
}
Variables and Operators
Variables in Python can be assigned values using the equal sign (=). You can then use these variables in your code to store and manipulate data. Here’s an example that demonstrates the use of variables and arithmetic operators:
x = 42
y = 7
z = x + y
print(z) # Output: 49
Python also supports various comparison operators (>, <, ==, etc.), and logical operators (and, or, not). Here’s an example that demonstrates the use of comparison and logical operators:
x = 42
y = 7
# Comparison
is_greater = x > y
print(is_greater) # Output: True
# Logical
is_true = (x > y) and (x != 0)
print(is_true) # Output: True
Functions and Loops
Python supports reusable code blocks through the use of functions. Functions can be defined using the def
keyword. Here’s an example of a function that calculates the area of a circle:
import math
def circle_area(radius):
return math.pi * radius * radius
radius = 5
area = circle_area(radius)
print(area) # Output: 78.53981633974483
Loops are used to repeat code blocks in Python. There are two common types of loops in Python: for
loops and while
loops. Here’s an example of a for
loop that outputs the numbers 1 to 10:
for i in range(1, 11):
print(i)
# Output:
# 1
# 2
# 3
# 4
# 5
# 6
# 7
# 8
# 9
# 10
And here’s an example of a while loop that calculates the sum of the first 10 positive integers:
i = 1
sum = 0
while i <= 10:
sum += i
i += 1
print(sum) # Output: 55
Introduction to Functions
Anatomy of a function
A function in Python is defined using the def keyword, followed by the name of the function, and a set of parentheses containing zero or more arguments. The body of the function is indented, and can contain one or more statements that define the behavior of the function.
Here’s the syntax for defining a function in Python:
def function_name(argument1, argument2, ...):
# function body
return result
The argument1, argument2, etc. are placeholders for the values that will be passed to the function when it is called. The return statement is used to return a value from the function.
Defining and calling a function
Here’s an example of how to define a function that adds two numbers together, and then call the function with two arguments:
def add(a, b):
return a + b
sum = add(2, 3) # sum = 5
In this example, the function is called add, and it takes two arguments, a and b. The function body contains a single statement that adds the two arguments together, and then returns the result.
To call the function, we pass two arguments to it, 2 and 3, which are assigned to the a and b parameters of the function. The result of the function, which is 5, is then assigned to the variable sum.
Parameters and arguments
A parameter is a variable in a function definition that is used to accept arguments that are passed to the function. An argument is a value that is passed to the function when it is called.
In Python, you can define default values for parameters. This means that if an argument is not provided for a parameter when the function is called, the default value will be used instead.
Here’s an example of a function that multiplies two numbers together, and uses a default value of “1” for the second parameter:
def multiply(a, b=1):
return a * b
product1 = multiply(2, 3) # product1 = 6
product2 = multiply(2) # product2 = 2
In this example, the function is called multiply, and it takes two parameters, a and b. The second parameter, b, has a default value of 1.
When the function is called with two arguments, 2 and 3, the values 2 and 3 are assigned to the a and b parameters, and the function returns the product of the two numbers, which is 6.
When the function is called with only one argument, 2, the value 2 is assigned to the a parameter, and the default value of 1 is used for the b parameter. The function returns the product of the two numbers, which is 2.
Advanced Function Concepts
Default arguments
In Python, you can define default values for function parameters. This means that if an argument is not provided for a parameter when the function is called, the default value will be used instead.
Here’s an example of a function that displays information about a person, with a default value of ‘unknown’ for the gender parameter:
def display_info(name, age, gender='unknown'):
print('Name:', name)
print('Age:', age)
print('Gender:', gender)
display_info('John', 25)
In this example, the function is called display_info, and it takes three parameters, name, age, and gender. The gender parameter has a default value of ‘unknown’.
When the function is called with two arguments, John and 25, the values John and 25 are assigned to the name and age parameters, and the default value of ‘unknown’ is used for the gender parameter. The function prints out the information about the person, including the default value of ‘unknown’ for the gender.
Keyword arguments
In Python, you can use keyword arguments to pass arguments to a function by name, rather than by position. This can make the code more readable and easier to understand, especially when there are many arguments.
Here’s an example of a function that displays information about a person using keyword arguments:
def display_info(name, age, gender='unknown'):
print('Name:', name)
print('Age:', age)
print('Gender:', gender)
display_info(age=25, name='John')
In this example, the function is called display_info, and it takes three parameters, name, age, and gender. The gender parameter has a default value of ‘unknown’.
When the function is called with two keyword arguments, age=25 and name=’John’, the values John and 25 are assigned to the name and age parameters, and the default value of ‘unknown’ is used for the gender parameter. The function prints out the information about the person, using the values passed in as keyword arguments.
Variable-length arguments
In Python, you can use the *args syntax to pass a variable number of arguments to a function. This is useful when you don’t know how many arguments a function will receive, or when you want to accept a variable number of arguments.
Here’s an example of a function that takes a variable number of arguments and returns their sum:
def add(*args):
sum = 0
for arg in args:
sum += arg
return sum
total = add(1, 2, 3, 4, 5) # total = 15
In this example, the function is called add, and it takes a variable number of arguments using the *args syntax. The function body uses a for loop to iterate over the arguments, and adds them up to get the total sum. The function returns the sum.
When the function is called with the arguments 1, 2, 3, 4, 5, the function adds them up and returns the sum, which is 15.
Scope of variables
In Python, the scope of a variable refers to where the variable is defined and where it can be accessed from. Variables can have either global or local scope.
Global variables are defined outside of any function, and can be accessed from anywhere in the program. Local variables are defined inside a function, and can only be accessed within that function.
Here’s an example of a function that demonstrates the scope of variables:
def add(a, b):
total = a + b # local variable
return total
a = 5 # global variable
b = 10 # global variable
total = add(a, b)
print(total) # prints 15
print(a) # prints 5
print(b) # prints 10
print(total) # prints 15
In this example, the function is called add, and it calculates the sum of two numbers a and b, and assigns the result to the total variable, which is a local variable. The function returns the total value.
The program also defines two global variables, a and b, which have values of 5 and 10, respectively. The add function is called with the a and b values, and the result is assigned to a global variable called total.
The program then prints the total value, which is 15, as well as the a and b values, which are still 5 and 10. This demonstrates that the total variable is a local variable and can only be accessed within the add function.
Lambda functions
Lambda functions are anonymous functions that can be defined inline. Here’s an example:
square = lambda x: x * x
print(square(5)) # Output: 25
In this example, lambda x: x * x is a lambda function that takes a single argument x and returns its square.
Map and filter functions
The map and filter functions are built-in functions that can be used to apply functions to sequences of data. Here are some examples:
# Map function
numbers = [1, 2, 3, 4, 5]
squares = map(lambda x: x * x, numbers)
print(list(squares)) # Output: [1, 4, 9, 16, 25]
# Filter function
numbers = [1, 2, 3, 4, 5]
even_numbers = filter(lambda x: x % 2 == 0, numbers)
print(list(even_numbers)) # Output: [2, 4]
In these examples, map and filter are used to apply a lambda function to a sequence of data.
List comprehensions
List comprehensions are a concise way to create lists based on existing lists. Here’s an example:
numbers = [1, 2, 3, 4, 5]
squares = [x * x for x in numbers]
print(squares) # Output: [1, 4, 9, 16, 25]
In this example, a new list squares is created by applying the x * x function to each element in the numbers list.
Higher-order functions
A higher-order function is a function that takes one or more functions as arguments, or returns a function as its result. Here’s an example:
def apply_twice(func, arg):
return func(func(arg))
def add_five(x):
return x + 5
print(apply_twice(add_five, 10)) # Output: 20
In this example, apply_twice is a higher-order function that takes a function func and an argument arg. It applies the func function to the arg argument twice.
Modules and Packages
Modules
In Python, a module is a file that contains a collection of related functions and variables that can be used in other programs. You can import modules into your Python programs to use their functionality.
Here’s an example of a module that defines a function that returns the factorial of a number:
# math.py
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
In this example, the math.py file defines a function called factorial that takes an integer n as an argument and returns the factorial of n. The function uses recursion to calculate the factorial.
To use this module in another Python program, you can import it using the import statement:
import math
result = math.factorial(5) # result = 120
In this example, the math module is imported using the import statement. The factorial function is then called with the argument 5, and the result is assigned to a variable called result.
Packages
In Python, a package is a collection of related modules that are organized in a directory hierarchy. Packages can contain subpackages and modules, and can be used to organize large Python projects.
Here’s an example of a package that contains two modules, math.py and statistics.py:
mypackage/
__init__.py
math.py
statistics.py
The init.py file is a special file that is executed when the package is imported. The other files contain the functions and variables that are part of the package.
To use this package in another Python program, you can import the modules using the import statement:
import mypackage.math
import mypackage.statistics
result = mypackage.math.factorial(5) # result = 120
mean = mypackage.statistics.mean([1, 2, 3, 4, 5]) # mean = 3.0
In this example, the mypackage package is imported using the import statement. The math and statistics modules are then imported using the package name and module name separated by a dot. The factorial function from the math module is called with the argument 5, and the result is assigned to a variable called result. The mean function from the statistics module is called with a list of numbers, and the result is assigned to a variable called mean.
Advanced data structures and algorithms
Sets
Sets are a collection of unique elements that can be used to perform set operations such as union, intersection, and difference. Here’s an example:
set1 = set([1, 2, 3, 4, 5])
set2 = set([4, 5, 6, 7, 8])
# Union
print(set1.union(set2)) # Output: {1, 2, 3, 4, 5, 6, 7, 8}
# Intersection
print(set1.intersection(set2)) # Output: {4, 5}
# Difference
print(set1.difference(set2)) # Output: {1, 2, 3}
In this example, set1 and set2 are sets that can be used to perform set operations such as union, intersection, and difference.
Heaps
A heap is a data structure that allows for efficient retrieval of the smallest or largest element in a collection. Here’s an example:
import heapq
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
# Convert list to heap
heapq.heapify(numbers)
# Retrieve smallest elements
print(heapq.heappop(numbers)) # Output: 1
print(heapq.heappop(numbers)) # Output: 1
# Add element to heap
heapq.heappush(numbers, 0)
# Retrieve smallest elements again
print(heapq.heappop(numbers)) # Output: 0
print(heapq.heappop(numbers)) # Output: 2
In this example, heapq is a Python module that can be used to perform heap operations on a list of numbers.
Tries
A trie is a tree-like data structure that is used to store strings. Each node in the trie represents a prefix of one or more strings. Here’s an example:
class Trie:
def __init__(self):
self.root = {}
def add_word(self, word):
current = self.root
for letter in word:
current = current.setdefault(letter, {})
current.setdefault("_end")
def contains_word(self, word):
current = self.root
for letter in word:
if letter not in current:
return False
current = current[letter]
return "_end" in current
In this example, Trie is a class that represents a trie data structure. The add_word method adds a word to the trie, and the contains_word method checks if a word is in the trie.
Graphs
A graph is a collection of nodes and edges that can be used to represent complex relationships between data. Here’s an example:
class Graph:
def __init__(self):
self.nodes = {}
def add_node(self, node):
self.nodes[node] = set()
def add_edge(self, node1, node2):
self.nodes[node1].add(node2)
self.nodes[node2].add(node1)
In this example, Graph is a class that represents an undirected graph. The add_node method adds a node to the graph, and the add_edge method adds an edge between two nodes in the graph.
Concurrency and Parallelism
Threading
Threading is a way to execute multiple threads (smaller units of code) simultaneously within a single process. Here’s an example:
import threading
def count():
for i in range(1, 11):
print(i)
def main():
t1 = threading.Thread(target=count)
t2 = threading.Thread(target=count)
t1.start()
t2.start()
t1.join()
t2.join()
if __name__ == '__main__':
main()
In this example, the count function is run in two separate threads, t1 and t2. The start method is called on each thread to begin executing them simultaneously. The join method is called on each thread to wait for it to finish executing before continuing.
Multiprocessing
Multiprocessing is a way to execute multiple processes (separate instances of a program) simultaneously. Here’s an example:
import multiprocessing
def count():
for i in range(1, 11):
print(i)
def main():
p1 = multiprocessing.Process(target=count)
p2 = multiprocessing.Process(target=count)
p1.start()
p2.start()
p1.join()
p2.join()
if __name__ == '__main__':
main()
In this example, the count function is run in two separate processes, p1 and p2. The start method is called on each process to begin executing them simultaneously. The join method is called on each process to wait for it to finish executing before continuing.
Asyncio
Asyncio is a way to write asynchronous code using coroutines, which are functions that can be paused and resumed. Here’s an example:
import asyncio
async def count():
for i in range(1, 11):
print(i)
await asyncio.sleep(1)
async def main():
await asyncio.gather(count(), count())
if __name__ == '__main__':
asyncio.run(main())
In this example, the count coroutine is run twice simultaneously using the gather method. The await keyword is used to pause the coroutine when it is waiting for the sleep method to complete.
Error Handling in Python
Error handling is an important part of any programming language, and Python is no exception. Python provides a rich set of tools for dealing with errors, from basic exception handling to more advanced techniques such as context managers and decorators.
Handling Exceptions with try-except
The most basic form of error handling in Python is the try-except block. This allows you to catch and handle exceptions that might be raised by your code.
Here’s an example of using a try-except block to handle a ZeroDivisionError:
try:
result = 1 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
In this example, we’re trying to divide 1 by 0, which would normally raise a ZeroDivisionError. But we’re using a try-except block to catch the error and print a custom error message instead.
You can also catch multiple types of exceptions in the same block:
try:
# some code that might raise an exception
except (ExceptionType1, ExceptionType2):
# handle exceptions of type ExceptionType1 or ExceptionType2
Raising Exceptions with raise
In addition to catching exceptions, you can also raise your own exceptions using the raise statement. This allows you to create custom error messages and handle them in your own code.
Here’s an example of raising a ValueError if a function is called with an invalid argument:
def my_function(arg):
if arg < 0:
raise ValueError("Argument must be non-negative")
# rest of the function
In this example, we’re checking if the arg parameter is negative, and raising a ValueError if it is. This allows us to catch the error and handle it in our own code.
Using Context Managers for Error Handling
Context managers are a powerful tool for managing resources in Python, and can also be used for error handling. A context manager is an object that defines methods to be called when a block of code is entered and exited.
One common use case for context managers is to ensure that resources such as files or network connections are properly closed when they are no longer needed. Here’s an example of using a context manager to open and close a file:
with open("file.txt", "r") as f:
# some code that reads from the file
In this example, we’re using the with statement to create a context manager for opening the file.txt file in read mode. The file will be automatically closed when the with block is exited, even if an error is raised.
Using Decorators for Error Handling
Decorators are another powerful tool in Python, and can be used for error handling as well. A decorator is a function that takes another function as its argument, and returns a new function that usually extends the behavior of the original function.
One common use case for decorators is to add error handling code to a function. Here’s an example of using a decorator to handle exceptions in a function:
def handle_errors(func):
def wrapper(*args, **kwargs):
try:
return func(*args, **kwargs)
except Exception as e:
print("Error:", e)
return wrapper
@handle_errors
def my_function(arg):
# some code that might raise an exception
In this example, we’re defining a decorator function handle_errors that takes a function as its argument and returns a new function that wraps the original function in a try-except block. If an exception is raised, the error message is printed to the console.
We’re then using the @handle_errors decorator to modify the behavior of the my_function function. Any exceptions raised by my_function will now be handled by the decorator.
Youtube / Video Tutorials
There are many excellent tutorials available on YouTube, such as:
- Python Tutorial – Python Full Course for Beginners by Programming with Mosh states Go from Zero to Hero with Python (includes machine learning & web development projects). This course covers everything from the very basics of Python syntax to building real-world applications using Python.
- Python for Beginners – Full Course is a comprehensive programming tutorial created by FreeCodeCamp.org that is designed to teach the basics of Python to beginners. This course is ideal for people who have no prior programming experience, and it covers everything from setting up the development environment to building real-world applications.
- Learning to program with Python 3 (py 3.7) by Sentdex. These videos are a great way to get a feel for the language and start writing your own code.
Reference Documentation and Forums
The Python reference documentation is an extensive resource for all things related to the Python programming language. The documentation can be found at https://docs.python.org/3/ and is maintained by the Python Software Foundation.
The documentation includes information on the Python standard library, which provides a large collection of modules and functions that can be used in Python programs. The documentation also covers the Python language itself, including syntax and semantics.
In addition to the standard library and language reference, the documentation includes information on how to use various tools and packages that are commonly used with Python, such as pip and virtual environments. It also includes information on how to extend Python with C or other programming languages.
The Python reference documentation is organized in a clear and logical manner, making it easy to navigate and find the information you need. It is an essential resource for anyone who is serious about learning and working with the Python programming language.
Blog Posts: There are many comprehensive blog posts available online, such as those found on Real Python, Python.org, and Towards Data Science. These can provide more in-depth information on specific topics and allow you to dive deeper into the language.
Discussion Forums : Here are some websites that offer both FAQ and forums specifically for Python code:
- Stack Overflow -Stack Overflow is a popular Q&A website for programming-related questions, including Python. They have a large community of active users who can help answer questions, and many questions related to Python have already been answered and can be found through searching.
- Reddit – The Python subreddit (/r/Python) is a popular community of Python developers who discuss various topics related to Python, including code debugging and sharing. The subreddit includes a FAQ section and is a good resource for finding answers to your Python-related questions.
- Python-forum.io – This is a community-driven forum where you can ask for help with your Python code, get feedback from other developers, and participate in discussions related to Python programming. They also have a FAQ section that covers a wide range of Python-related topics.
Technical Books
Here are a few technical books on Python, along with their authors, publishers, ISBN and description, I personally found usefull:
- “Learning Python, 5th Edition” by Mark Lutz, published by O’Reilly Media: This book is a comprehensive guide to programming with Python, covering topics such as data types, control structures, functions, and object-oriented programming. It is designed for beginners who are new to programming and want to learn Python from scratch. ISBN-13: 978-1449355739.
- “Python Crash Course, 2nd Edition: A Hands-On, Project-Based Introduction to Programming” by Eric Matthes, published by No Starch Press: This book is a hands-on guide to programming with Python, with a focus on project-based learning. It covers basic concepts such as data types, control structures, functions, and file handling, and also includes chapters on web development, game development, and data visualization. It is designed for beginners who want to learn Python through hands-on projects. ISBN-13: 978-1593279288.
- “Automate the Boring Stuff with Python: Practical Programming for Total Beginners, 2nd Edition” by Al Sweigart, published by No Starch Press: This book is a practical guide to using Python for automating tasks, such as file manipulation, web scraping, and more. It is designed for beginners who are new to programming and want to learn how to automate tasks with Python. ISBN-13: 978-1593279929.
- “Python Pocket Reference, 5th Edition” by Mark Lutz, published by O’Reilly Media: This book is a handy reference guide to Python, including syntax, built-in functions, and libraries. It is designed for developers who already have some experience with Python and want a quick reference guide. ISBN-13: 978-1492051367.
- “Python Cookbook, 3rd Edition” by David Beazley and Brian K. Jones, published by O’Reilly Media: This book is a collection of practical recipes for solving common programming problems in Python. It covers a wide range of topics, from data structures and algorithms to network programming and web development. It is designed for intermediate to advanced Python developers who want to expand their knowledge of the language. ISBN-13: 978-1449340377.
- “Python for Everybody: Exploring Data in Python 3” by Charles Severance, published by CreateSpace Independent Publishing Platform: This book is an introductory guide to programming with Python, focusing on data analysis and visualization. It is designed for beginners who are new to programming and want to learn how to use Python for data analysis. ISBN-13: 978-1530051120.
- “Python Data Science Handbook: Essential Tools for Working with Data” by Jake VanderPlas, published by O’Reilly Media: This book is a comprehensive guide to using Python for data science, including topics such as data manipulation, visualization, and machine learning. It is designed for developers who already have some experience with Python and want to learn how to use it for data science. ISBN-13: 978-1491912058.
- “Fluent Python: Clear, Concise, and Effective Programming” by Luciano Ramalho, published by O’Reilly Media: This book is an in-depth guide to advanced Python programming, including topics such as object-oriented programming, functional programming, and metaprogramming. It is designed for experienced Python developers who want to take their programming skills to the next level. The book features practical examples and exercises to help readers improve their Python programming skills. ISBN-13: 978-1491946008.
- “A Beginner’s Guide to Python 3 Programming” by John Hunt, published by Springer: This book is a beginner’s guide to programming with Python 3, designed for readers who have no prior experience with programming or Python. It covers basic concepts such as data types, control structures, functions, and file handling, and also includes a chapter on object-oriented programming. The book features numerous examples and exercises to help readers practice their skills. ISBN-13: 978-3030219854.
- “Advanced Guide to Python 3 Programming” by John Hunt, published by Springer: This book is an advanced guide to programming with Python 3, designed for readers who have prior experience with programming or Python. It covers advanced topics such as data structures, algorithms, concurrency, networking, and web development. The book features practical examples and exercises to help readers improve their Python programming skills. ISBN-13: 978-3030443244.
Cheat Sheets
Python Basics Cheatsheet:
The “Python Basics” cheatsheet from Real Python provides a quick reference for common data types, variables, operators, functions, and control structures in Python. It also includes tips for working with strings, lists, and dictionaries, as well as a section on common errors and how to avoid them.
Python Standard Library Cheatsheet:
The “Python” cheatsheet from Mosh Hamedani is a useful resource for accessing the vast number of modules available in the Python Standard Library. It includes information on modules for file I/O, data structures, math and science, and more, making it easy to find the right module for your needs.
Conclusion
The key to becoming proficient in Python is to keep practicing and experimenting with the language. Don’t be afraid to try new things and make mistakes – with persistence and practice, you’ll become a skilled Python programmer in no time.