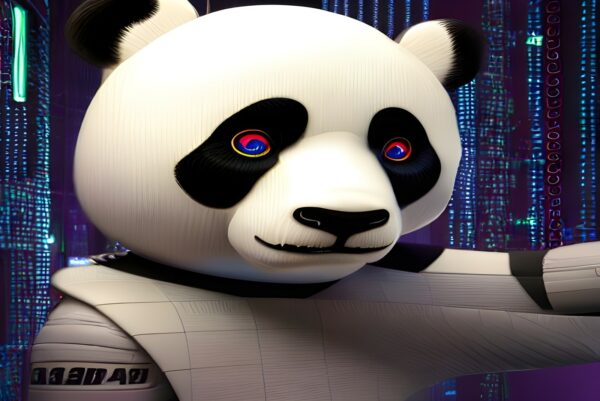
Pandas is a popular open-source data analysis and data manipulation library in Python. It provides data structures and functions needed to work with structured data seamlessly and efficiently. Pandas are fast, flexible, and powerful, making it the go-to library for many data scientists and data analysts worldwide.
In this article, we will introduce Pandas and its key features, provide a comprehensive training course for Pandas, and include code examples, YouTube videos, and technical references to help you get started with the library.
Introduction to Pandas
Pandas is a library that provides high-performance, easy-to-use data structures and data analysis tools for the Python programming language. It was developed by Wes McKinney in 2008 and has since become one of the most widely used libraries for data analysis and data processing.
One of the key features of Pandas is the DataFrame, a two-dimensional labeled data structure that can store and manipulate a large amount of data. The DataFrame is similar to a table in a relational database or a spreadsheet in Microsoft Excel. Pandas also provides other data structures like Series (a one-dimensional labeled array) and Panel (a three-dimensional data structure), but DataFrames are the most widely used.
With Pandas, you can easily read data from a variety of sources, including CSV files, Excel spreadsheets, SQL databases, and even from the web. You can also manipulate data with ease, such as filtering, sorting, aggregating, and transforming data. Pandas also provides functions for performing complex operations like merging, joining, and pivoting data.
Pandas Training Course
In this section, we’ll provide a comprehensive training course for Pandas, including code examples and step-by-step explanations.
- Installation
To use Pandas, you must first install it. You can install Pandas using the following command:
pip install pandas
- Importing Pandas
Once you have installed Pandas, you can import it into your Python script using the following code:
import pandas as pd
- Reading Data
Pandas provides several functions for reading data from different sources, including read_csv
, read_excel
, and read_sql
. Here is an example of how to read data from a CSV file using the read_csv
function:
data = pd.read_csv('data.csv')
- Exploring Data
Once you have read the data into a DataFrame, you can explore the data using various functions and attributes. Here are some of the most commonly used functions and attributes:
- head: returns the first n rows of the DataFrame
- tail: returns the last n rows of the DataFrame
- shape: returns the number of rows and columns of the DataFrame
- info: returns information about the DataFrame, including the number of non-null values, data types, and memory usage
- describe: returns descriptive statistics of the DataFrame
- Cleaning Data
Data is rarely clean and ready to use, and often requires cleaning and preprocessing. Pandas provides several functions for cleaning data, including:
- dropna: drops rows or columns with missing values
- fillna: fills missing values with a specified value or method
- replace: replaces values in the DataFrame
- astype: changes the data type of a column in the DataFrame
Here’s an example of how to use the fillna function to fill missing values in a DataFrame:
data = data.fillna(0)
- Manipulating Data
Pandas provides several functions for manipulating data, including:
- sort_values: sorts the DataFrame by one or more columns
- groupby: groups the data by one or more columns and aggregates the data using functions like mean, sum, count, etc.
- pivot: pivots the data to create a new DataFrame with columns as values and index as row labels
- merge: merges two DataFrames on one or more columns
- join: joins two DataFrames on the index
Here’s an example of how to use the groupby function to group data and calculate the mean of each group:
grouped_data = data.groupby('column_name').mean()
- Visualizing Data
Pandas provides integration with the matplotlib library, which is a popular library for data visualization in Python. You can use the plot
function in Pandas to quickly visualize your data.
Here’s an example of how to create a line plot of the data:
data.plot(kind='line')
Youtube / Video Tutorials
These are three great resources to learn Pandas, a popular Python library used for data analysis and manipulation. Here’s a brief overview of each tutorial:
- Pandas Tutorial – Full Course for Beginners by Corey Schafer: This tutorial is a comprehensive guide to learning Pandas, covering everything from the basics of data structures like series and dataframes, to advanced topics like merging and reshaping data. The tutorial is well-organized and structured, making it easy to follow along with the code examples. The instructor, Corey Schafer, is a well-known programming instructor with a clear teaching style and an engaging presentation.
- Pandas Tutorial (Data Analysis with Python) by codebasics: This tutorial is another great introduction to Pandas, focused on using it for data analysis. It covers topics like filtering, grouping, and aggregating data, as well as handling missing data and working with dates and times. The instructor, codebasics, has a practical teaching style that emphasizes working with real-world datasets.
- Introduction to Pandas for Data Analysis by Data School: This tutorial provides a thorough introduction to Pandas, starting with the basics of data structures and quickly moving into more advanced topics like pivot tables and multi-level indexing. The instructor, Kevin Markham, is a data scientist with a wealth of experience using Pandas and a clear, concise teaching style.
Overall, each of these tutorials offers a great way to get started with Pandas and become proficient in using it for data analysis. It’s worth noting that while all three tutorials cover similar material, each has its own unique approach and teaching style, so it may be worth watching multiple tutorials to get a more complete understanding of the library.Regenerate respon
Reference Documentation and Forums
Here are some helpful resources for finding reference documentation and forums for Pandas, including links:
- Pandas documentation: The official documentation for Pandas is a great resource for reference information, tutorials, and examples. It includes a user guide, API reference, and a wide range of tutorials and examples. Here is the link: https://pandas.pydata.org/docs/
- Pandas User Group: The Pandas User Group is a community of users who share knowledge and help each other with problems related to Pandas. It’s a great place to ask questions, share tips and tricks, and learn from others. Here is the link: https://groups.google.com/g/pandas-users
- Stack Overflow: Stack Overflow is a popular forum for programmers to ask and answer technical questions. There are many questions related to Pandas on Stack Overflow, and it’s often a great place to find solutions to specific problems. Here is the link: https://stackoverflow.com/questions/tagged/pandas
- GitHub: The Pandas project is hosted on GitHub, and you can find the source code, issues, and pull requests there. This can be a great resource for advanced users who want to contribute to the project or understand how it works under the hood. Here is the link: https://github.com/pandas-dev/pandas
- PyData: PyData is a community of users interested in data science and related technologies, including Pandas. The PyData website includes many resources for learning and using Pandas, including videos of talks from past conferences. Here is the link: https://pydata.org/
I hope these resources help you find the information you need to learn and use Pandas effectively!Regenerate response
Reference Technical Books
Here are some reference technical books for Pandas, including their author, publisher, ISBN, and description:
- Python for Data Analysis: Data Wrangling with Pandas, NumPy, and IPython by Wes McKinney, O’Reilly Media, ISBN: 978-1491957660. This book is written by the creator of Pandas, and it provides a comprehensive introduction to using Pandas for data analysis. It covers the basics of Pandas data structures, data wrangling and cleaning, time series analysis, and more.
- Pandas Cookbook by Theodore Petrou, Packt Publishing, ISBN: 978-1784393878. This book is a practical guide to using Pandas for data analysis. It includes a wide range of recipes for common data analysis tasks, such as data cleaning, data transformation, and data visualization.
- Pandas in Action by Boris Paskhaver, Manning Publications, ISBN: 978-1617296159. This book is a hands-on guide to using Pandas for data analysis. It covers the basics of Pandas data structures, data cleaning and transformation, time series analysis, and more. The book also includes practical examples and exercises to help you apply what you’ve learned.
- Mastering Pandas for Finance by Michael Heydt, Packt Publishing, ISBN: 978-1783985101. This book focuses on using Pandas for financial analysis, including working with time series data, calculating financial metrics, and backtesting trading strategies. It also covers advanced topics such as machine learning for financial modeling.
- Data Wrangling with Pandas by Kevin Markham, Packt Publishing, ISBN: 978-1788397504. This book provides a comprehensive introduction to using Pandas for data cleaning and manipulation. It covers topics such as merging and reshaping data, working with missing data, and creating new variables.
Overall, these books provide a range of resources for learning and using Pandas effectively, whether you’re new to the library or an experienced user looking to deepen your knowledge.
Cheat Sheet
If you’re working with data in Python, the “Pandas” cheatsheet from DataCamp is a must-have resource. It provides a comprehensive overview of the Pandas library, including information on data frames, series, and data analysis techniques. Whether you’re a beginner or an experienced data analyst, this cheatsheet will help you work with data more efficiently.
Conclusion
Pandas is a powerful and versatile library for data analysis and processing in Python. Whether you’re a beginner or an experienced data analyst, Pandas has everything you need to work with structured data effectively and efficiently. We hope this article and training course have been helpful in getting you started with Pandas. Good luck and happy data processing!